纯html,js,css做最简单的Flappy Bird小游戏
效果:
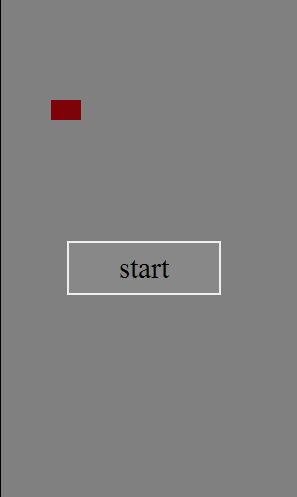
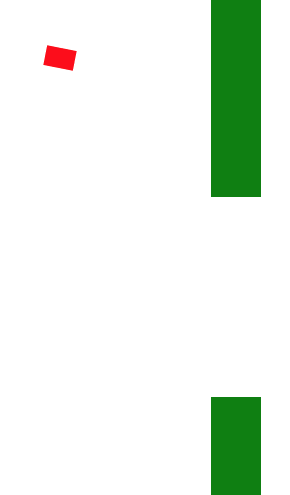
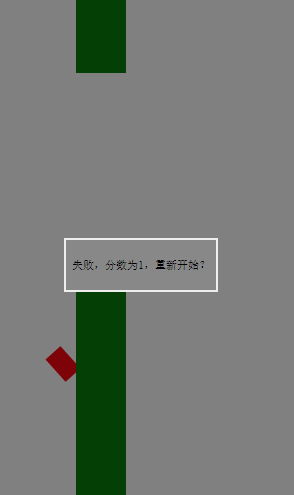
主要使用了定时器,做出速度与加速度的效果,并配合改变Bird的角度,达到想要的效果。
检测游戏是否失败靠检测鸟的上下左右点是否在柱子内或者在地图外。
Html: 1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
<html>
<head>
<meta charset="utf-8" />
<title></title>
</head>
<body>
<div id="start" onclick="start()">start</div>
<div id="startbackground"></div>
<div id="warp" onclick="tap()">
<div id="bird"></div>
<div id="pillar-up"></div>
<div id="pillar-down"></div>
<div id="background"></div>
</div>
</body>
<style>
body{
height: 500px;
width: 300px;
}
#start {
position: fixed;
z-index: 999;
top: 250px;
left: 150px;
border: 2px solid#eee;
background-color: #888;
height: 50px;
width: 150px;
margin-left: -75px;
text-align: center;
line-height: 50px;
font-size: 30px;
}
#startbackground {
position: fixed;
z-index: 888;
height: 500px;
width: 300px;
background-color: black;
opacity: 0.5;
}
#warp {
position: relative;
border: 1px solid#000;
height: 500px;
width: 300px;
}
#bird {
position: absolute;
height: 20px;/*鸟高 */
width: 30px;/*鸟长*/
background-color: red;
top: 100px;
left: 50px;
transform: rotate(0deg);
-ms-transform: rotate(0deg);
-moz-transform: rotate(0deg);
-webkit-transform: rotate(0deg);
-o-transform: rotate(0deg);
}
#pillar-up {
position: absolute;
height: 0;
width: 50px;
background-color: green;
top: 0;
right: -50px;
}
#pillar-down {
position: absolute;
height: 0;
width: 50px;
background-color: green;
bottom: 0;
right: -50px;
}
</style>
<script>
var startX = 50;//角色距离左边x坐标
var startY = 100; //角色开始高度
var maxTop = 480; //地图高度500 角色碰撞高度20
var v_y = 0; //向下运动为正,每1个时间单位向下移动v_y个像素
var v_x = 2; //水平相对运动速度(实际上没有运动)
var g = 0.7; //重力加速度,每个时间单位速度向下加这么多
var Bird = document.getElementById("bird");
var startBtn = document.getElementById("start");
var pillarup = document.getElementById("pillar-up");
var pillardown = document.getElementById("pillar-down");
var pillarX = -50; //柱子初始位置
var gamestate = 0;
var pillarstate = 0;
var scorecount = 0;
var score = 0;//分数
function start() {
if (gamestate == 1) {
window.location.reload()
} else {
main();
startBtn.style.display = "none";
document.getElementById("startbackground").style.display = "none";
}
}
function tap() {
v_y = -12;
}
function main() {
var birdtimer = setInterval(function() {
if (startY > maxTop || startY < 0) { //角色碰撞上下边界
gameover();
}
if ((pillarX>220&&pillarX<280&&startY<pillarupH)||(pillarX>220&&pillarX<280&&startY>(200+ pillarupH))){
gameover();
}
startY += v_y;
v_y += g;
deg = Math.atan(v_y / v_x) / 1.57 * 60;
Bird.style.webkitTransform = "rotate(" + deg + "deg)";
Bird.style.MozTransform = "rotate(" + deg + "deg)";
Bird.style.msTransform = "rotate(" + deg + "deg)";
Bird.style.OTransform = "rotate(" + deg + "deg)";
Bird.style.transform = "rotate(" + deg + "deg)";
Bird.style.top = startY + "px";
}, 20) //一次时间单位
var pillartimer = setInterval(function() {
if (pillarstate == 0) {
pillarup.style.right = "-50px";
pillardown.style.right = "-50px";
pillarupH = 300 * Math.random();
pillarup.style.height = pillarupH + "px";
pillardown.style.height = (300 - pillarupH) + "px";
pillarstate = 1;
}
if (pillarX >= 350) {
pillarX = -50;
pillarstate = 0;
scorecount = 0;
}
if(pillarX >= 250&&scorecount == 0){
score++;
scorecount = 1;
}
pillarX += v_x;
pillarup.style.right = pillarX - 50 + "px";
pillardown.style.right = pillarX - 50 + "px";
}, 20) //一次时间单位
function gameover() {
clearTimeout(birdtimer);
clearTimeout(pillartimer);
document.getElementById("startbackground").style.display = "block"
startBtn.innerText = '失败,分数为'+ score +',重新开始?';
startBtn.style.display = 'block';
startBtn.style.fontSize = '11px';
gamestate = 1;
}
}
</script>
</html>